일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- 메탈퍼즐
- 발더스게이트
- 눈알빠지겠네
- DIY
- 취미
- 블라인드박스
- 노노그램
- 포켓몬
- 30mf
- 게임
- 누룽지소금빵
- 미앤아이
- 바질토마토뭐시기
- 코딩테스트
- 제프딕슨
- 프라모델
- 롱라이플
- 지쿠악스
- 건담
- 맛집
- LeetCode
- 건담헤드
- 지리데칼
- 가챠
- 밥무하마드
- 코테
- javascript
- 발더스모드
- 유루건
- 우리시대의역설
- Today
- Total
.Zzumbong
[leetCode/JS] 2038. Remove Colored Pieces if Both Neighbors are the Same Color 본문
[leetCode/JS] 2038. Remove Colored Pieces if Both Neighbors are the Same Color
쭘봉 2023. 10. 2. 18:42난이도 [ 🤔 ] Medium
문제 설명
There are n
pieces arranged in a line, and each piece is colored either by 'A'
or by 'B'
. You are given a string colors
of length n where colors[i]
is the color of the ith
piece.
Alice and Bob are playing a game where they take alternating turns removing pieces from the line. In this game, Alice moves first.
Alice is only allowed to remove a piece colored 'A'
if both its neighbors are also colored 'A'
. She is not allowed to remove pieces that are colored 'B'
.
Bob is only allowed to remove a piece colored 'B'
if both its neighbors are also colored 'B'
. He is not allowed to remove pieces that are colored 'A'
.
Alice and Bob cannot remove pieces from the edge of the line.
If a player cannot make a move on their turn, that player loses and the other player wins.
Assuming Alice and Bob play optimally, return true
if Alice wins, or return false
if Bob wins.
n개의 조각이 배열되어 있고, 각 조각은 'A' 또는 'B'로 색이 지정되어 되어 있음.
길이 n의 문자열 색상이 주어지며, 앨리스와 밥은 번갈아 가며 조각을 제거하는 게임을 한다.
앨리스가 항상 먼저 움직임. 앨리스는 'A' 조각을 제거할 수 있는데, 이 경우 이웃한 조각도 모두 'A' 색일 때만 가능
밥은 이웃도 모두 'B'인 경우에만 'B' 색의 조각을 제거할 수 있다.
앨리스와 밥은 선의 가장자리에서 조각을 제거할 수 없다.
한 플레이어가 자신의 차례에 이동을 하지 못하면, 그 플레이어는 패배하고 다른 플레이어가 승리.
앨리스와 밥이 최적으로 플레이한다고 가정하고, 앨리스가 이기면 true 반환하고, 밥이 이기면 false를 반환
입출력 예
Example 1:
Input: colors = "AAABABB"
Output: true
Explanation:
AAABABB -> AABABB
Alice moves first.
She removes the second 'A' from the left since that is the only 'A' whose neighbors are both 'A'.
Now it's Bob's turn.
Bob cannot make a move on his turn since there are no 'B's whose neighbors are both 'B'.
Thus, Alice wins, so return true.
Example 2:
Input: colors = "AA"
Output: false
Explanation:
Alice has her turn first.
There are only two 'A's and both are on the edge of the line, so she cannot move on her turn.
Thus, Bob wins, so return false.
Example 3:
Input: colors = "ABBBBBBBAAA"
Output: false
Explanation:
ABBBBBBBAAA -> ABBBBBBBAA
Alice moves first.
Her only option is to remove the second to last 'A' from the right.
ABBBBBBBAA -> ABBBBBBAA
Next is Bob's turn.
He has many options for which 'B' piece to remove. He can pick any.
On Alice's second turn, she has no more pieces that she can remove.
Thus, Bob wins, so return false.
Constraints
1 <= colors.length <= 105
colors
consists of only the letters'A'
and'B'
내 솔루션
- AAA가 있으면 Alice count에 +1, BBB가 있으면 Bob count에 +1을 해서 비교 후 누가 이기는지 알 수 있음.
/**
* @param {string} colors
* @return {boolean}
*/
var winnerOfGame = function(colors) {
let Acount = 0;
let Bcount = 0;
for(let i = 2; i < colors.length; i++) {
if(colors[i-2] === 'A' && colors[i-1] === 'A' && colors[i] === 'A'){
Acount++;
} else if (colors[i-2] === 'B' && colors[i-1] === 'B' && colors[i] === 'B'){
Bcount++;
}
}
return Acount > Bcount
};
// A나 B를 지우다보면 다시 지울 수 있는 놈이 생기지 않을까?
// 그래서 colors 자체를 다시 검사 해야하나? 라고 생각해서 재귀로 풀게됨.
// 너무 오래 걸려서 탈락
// const remove = (turn, colors) => {
// const index = colors.indexOf(turn === 'A' ? 'AAA' : 'BBB')
// if(index === -1) return turn === 'A' ? false : true
// return remove(turn === 'A' ? 'B' : 'A', colors.substring(0,index)+
// colors.substring(index+1, colors.length))
// }
// return remove('A', colors)
감상평
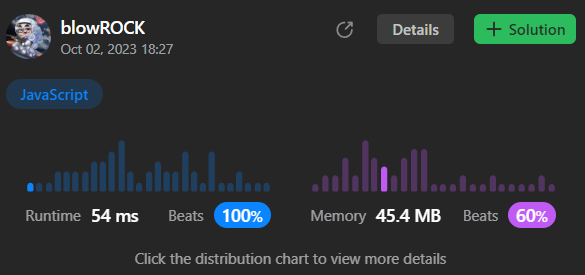
- 100% 처음 나왔다 신기방기
- 문제 자체가 좀 길어서 Medium 등급인가?
- 약간 헤매긴 했는데, 다른 leetcode나 알고리즘 테스트에 비해 쉬운 문제
'coding test > leetCode' 카테고리의 다른 글
[leetCode/JS] 6. Zigzag Conversion (0) | 2023.10.04 |
---|---|
[leetCode/JS] 1512. Number of Good Pairs (0) | 2023.10.03 |
[leetCode/JS] 557. Reverse Words in a String III (0) | 2023.10.01 |
[leetCode/JS] 1624. Largest Substring Between Two Equal Characters (0) | 2023.09.29 |
[leetCode/JS] 896. Monotonic Array (0) | 2023.09.29 |